Arithmetic operators are fundamental building blocks of programming, enabling us to perform calculations and manipulate numerical data.
In the realm of Arduino programming, arithmetic operators play a crucial role in controlling actuators, processing sensor data, and performing various computations essential for a wide range of projects.
This comprehensive guide delves into the world of Arduino arithmetic operators, providing a thorough explanation of each operator, its usage, and real-world examples.
For calculating arithmetic questions like addition, subtraction, multiplication or division we use arithmetic operators.
Arduino also can do mathematics for us. Yes, we also code the Arduino to find the solution to our arithmetic problems.
In this tutorial, we will learn how to do addition, subtraction, multiplication, and division.
Also, we will learn about how to use Float Variable, Modulo, Square Root and Number Power in Arduino. So Let’s begin.
Here some more tutorials for you:
- Getting started with Arduino.
- Arduino String Functionality.
- Arduino Generating Random Numbers.
- Serial communication in Arduino.
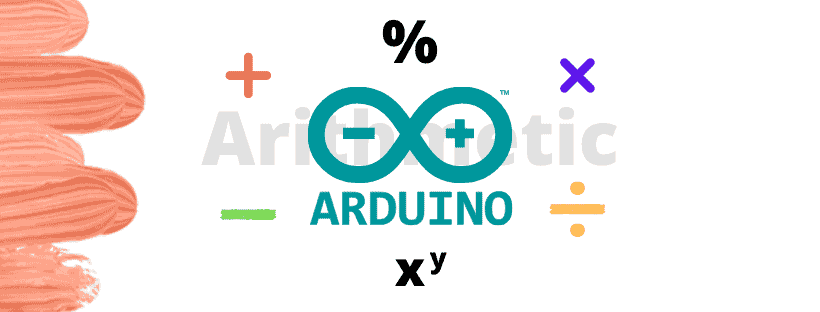
Addition
To add two or more numbers we use addition (+) operator. The following example shows how to add two numbers.
int a = 2;
int b = 3;
int sum;
sum = a + b;
In the above sketch, the three variables are defined as a, b, and sum. Variables a and b assigned an integer value 2 and 3 respectively.
The result of a + b will be stored in the sum variable. After the code execution sum will contain the value 5.
We also add two constant values and store the result in a variable.
int sum;
sum = 2 + 3;
The result stored in the sum variable and after execution, the statement will be 5.
Subtraction
To subtract numbers we use subtraction (-) operator. The following example shows how to subtract two numbers.
int a = 5;
int b = 3;
int subtract;
subtract = a - b;
int subtract;
subtract = a - b;
Multiplication
To multiply the numbers we use the multiplication (*) operator. The following example shows how to multiply two numbers.
int multiply;
multiply = 6 * 3;
The result of the above sketch is 18.
Division
To divide the numbers we use the division ( / ) operator. The following example shows how to division two numbers.
int divide;
divide = 10 / 2;
The result of the above sketch is 5.
Integer vs Float
In the above explanation, we have only used integer values. If we divide an odd number by an even number and it gives a fraction result than the integer variable will omit the fraction part and the result will show only an integer.
Let’s see an example of an integer variable:
int result;
result = 3 / 2;
The above sketch gives us 1 as a result but the actual result should be 1.5.
Now let’s see an example of a float variable:
float result;
result = 3.0 / 2.0;
Now the above sketch gives us an actual result 1.5.
Always use a decimal point for the whole number if you want a result in float i.e. 3.0 instead of 3.
Remainder (Modulo Division)
The remainder of a division is finding by using the remainder (%) operator.
int result;
result = 10 % 4;
The result of the above sketch is 2.
Raising a Number to a power (xy)
If we have to calculate a square or cube or any power of a number we use called it the number raised to a power.
Pow(x,y) returns the value of x raised to the power of y i.e. xy.
int result;
result = pow(3,2);
The above sketch calculates 32, so the result will be 9.
Square Root
To calculate the square root of any number we use sqrt() function. The following sketch will show the square root of 4.
Serial.println(sqrt(5));
The result of the above sketch is 2.24.
Arithmetic Code for All
The following sketch is for the whole explanation of the above article on the arithmetic operator.